As of Livebase version 5.6.2, the GraphQL schema provides services to invoke FormActionHandler and manage its return message. You can invoke a FormActionHandler by passing the service a form in a Create, Update or Save (generic writing) context, among the scenarios described in writing services.
The services described on this page are all of type mutation and respect the format <ClassName>___<scenario>___formAction___<HandlerName>
; these are generated for each FormActionHandler modeled on the class.
Reference model #
All examples on this page refer to the Employee class in the figure, and in particular, to the calculateSalary Handler circled in red:
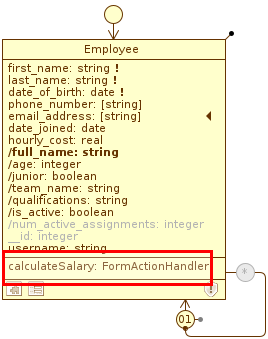
The Employee class, as modeled in the Tutorial, to which the Handler calculateSalary has been added.
This allows you to calculate the employee’s salary according to different criteria and automatically update the value of the hourly_cost attribute.
Detail: implementation
create___formAction
service #
The Mutation.<ClassName>___create___formAction___<HandlerName>
service allows you to invoke a FormActionHandler by passing the form context of create a new object of a certain class.
type Mutation {
ClassName___create___formAction___HandlerName(
data: ClassNameDraftCreate
jsonParam: String
forceWarnings: ForceWarnings
): ClassNameFormActionHandlerResult
}
The service accepts as input the structure <ClassName>DraftCreate
and returns as output the structure <ClassName>FormActionResult
. You can pass additional information to the Handler (serialized in JSON) via the jsonParam: String
field. Note that the date
field, as of Livebase version 5.11.2, is also optional and may not be valued in the mutation.
You can work around any warnings returned by the server caused by non-blocking Class Warnings by including the forceWarnings
argument, of type ForceWarnings
.
For example, the service to invoke the calculateSalary Handler of Employee in a Create context is as follows:
type Mutation {
Employee___create___formAction___calculateSalary(
data: EmployeeDraftCreate
jsonParam: String
forceWarnings: ForceWarnings
): EmployeeFormActionHandlerResult
}
update___formAction
service #
The Mutation.<ClassName>___update___formAction___<HandlerName>
service allows you to invoke a FormActionHandler by passing the form context of modifying an existing object of a certain class.
type Mutation {
ClassName___update___formAction___HandlerName(
data: ClassNameDraftUpdate
jsonParam: String
forceWarnings: ForceWarnings
): ClassNameFormActionHandlerResult
}
The service accepts the structure <ClassName>DraftUpdate
as input, and returns the structure <ClassName>FormActionResult
as output. You can pass additional information to the Handler (serialized in JSON) via the jsonParam: String
field. Note that the date
field, as of Livebase version 5.11.2, is also optional and may not be valued in the mutation.
You can work around any warnings returned by the server caused by non-blocking Class Warnings by including the forceWarnings
argument, of type ForceWarnings
.
For example, the service to invoke the calculateSalary Handler of Employee in an Update context is as follows:
type Mutation {
Employee___update___formAction___calculateSalary(
data: EmployeeDraftUpdate
jsonParam: String
forceWarnings: ForceWarnings
): EmployeeFormActionHandlerResult
}
formAction
service #
The Mutation.<ClassName>___formAction___<HandlerName>
service allows you to invoke a FormActionHandler by passing the context of a generic writing form over a certain class.
type Mutation {
ClassName___formAction___HandlerName(
data: ClassNameDraft
jsonParam: String
forceWarnings: ForceWarnings
): ClassNameFormActionHandlerResult
}
The service accepts as input the structure <ClassName>Draft
and returns as output the structure <ClassName>FormActionResult
. You can pass additional information to the Handler (serialized in JSON) via the jsonParam: String
field. Note that the date
field, as of Livebase version 5.11.2, is also optional and may not be valued in the mutation.
You can work around any warnings returned by the server caused by non-blocking Class Warnings by including the forceWarnings
argument, of type ForceWarnings
.
For example, the service to invoke the calculateSalary Handler of Employee in a Create or Update context is as follows:
type Mutation {
Employee___formAction___calculateSalary(
data: EmployeeDraft
jsonParam: String
forceWarnings: ForceWarnings
): EmployeeFormActionHandlerResult
}
FormActionHandler with Form classes in the input #
For FormActionHandlers to which a Form class is assigned (Set form...
on context menu of FormActionHandler, as described in reference on Form classes and Plugin development guide), an additional argument is generated in GraphQL services: formParam
, whose type is the input structure relative to the Form class pointed to by the Handler. This argument, when present, is required.
Depending on the context of the service (Create or generic write, respectively), the type of formParam
may be:
- for
create___formAction
:<FormClassName>DraftCreate
, analogous to<ClassName>DraftCreate
; - for generic
formAction
:<FormClassName>Draft
, analogous to<ClassName>Draft
.
For example, the signature of the generic formAction
service becomes the following:
type Mutation {
ClassName___formAction___HandlerName(
data: ClassNameDraft
formParam: FormClassNameDraft!
jsonParam: String
forceWarnings: ForceWarnings
): ClassNameFormActionHandlerResult
}
type <ClassName>FormActionHandlerResult
#
All the services mentioned so far return the following structure <ClassName>FormActionHandlerResult
:
type ClassNameFormActionHandlerResult {
data: ClassName # current object on which the handler has been invoked, possibly altered by plugin code
result: FormActionHandlerResult!
}
The data
field contains the structure <ClassName>
; this represents the current object on which the FormActionHandler was invoked, possibly altered by the Plugin code.
The result
field contains a common, model-independent structure of type FormActionHandlerResult
. By expanding this field, it is possible to obtain information about the result of the Handler invocation, which, as described here, can return an informational message, a warning message, or an error message.
type FormActionHandlerResult {
# analogous to the structure returned by the generated SPI FormActionHandler
type: FormActionHandlerResultType!
message: FormActionHandlerMessageResult
}
enum FormActionHandlerResultType {
MESSAGE
NONE
}
type FormActionHandlerMessageResult {
type: MessageType!
message: String!
title: String
}
enum MessageType {
ERROR
INFO
WARNING
}